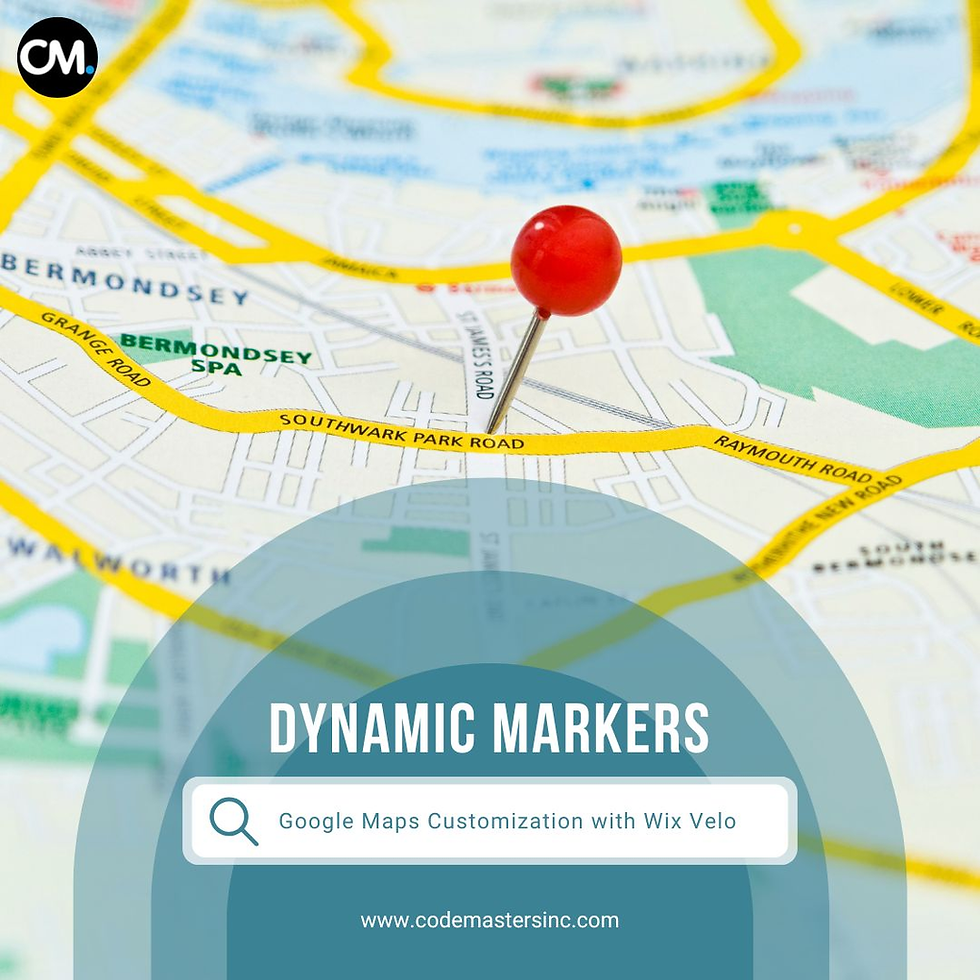
In the first part of our guide, we walked you through embedding Google Maps on your Wix website. While embedding a map can help visitors locate your business or venue easily, there’s much more you can do with Google Maps when paired with the power of Wix Velo. As Wix's full-stack development platform, Wix Velo enables advanced Google Maps customization, unlocking interactive features and dynamic mapping capabilities that elevate the user experience.
In this blog, we’ll explore how to integrate Google Maps with Wix Velo to display dynamic locations from your database, recenter the map to specific areas like New York, and add interactive markers that enhance user engagement. Let’s dive into the steps!
Step 1: Setting Up Your Google Maps API Key
Before we explore advanced features, ensure you’ve enabled the necessary APIs for your Google Cloud account. These include:
Maps JavaScript API
Geocoding API
Places API
If you haven’t done this yet, revisit the steps from Part 1. Once your API key is ready, move on to integrating it with Wix Velo.
Step 2: Adding a Google Map to Your Wix Page
Here’s how to add a Google Map with Wix Velo:
Add an HTML Component:
In the Wix Editor, go to Add (+) > Embed > HTML iframe.
Resize the iframe to fit your page layout.
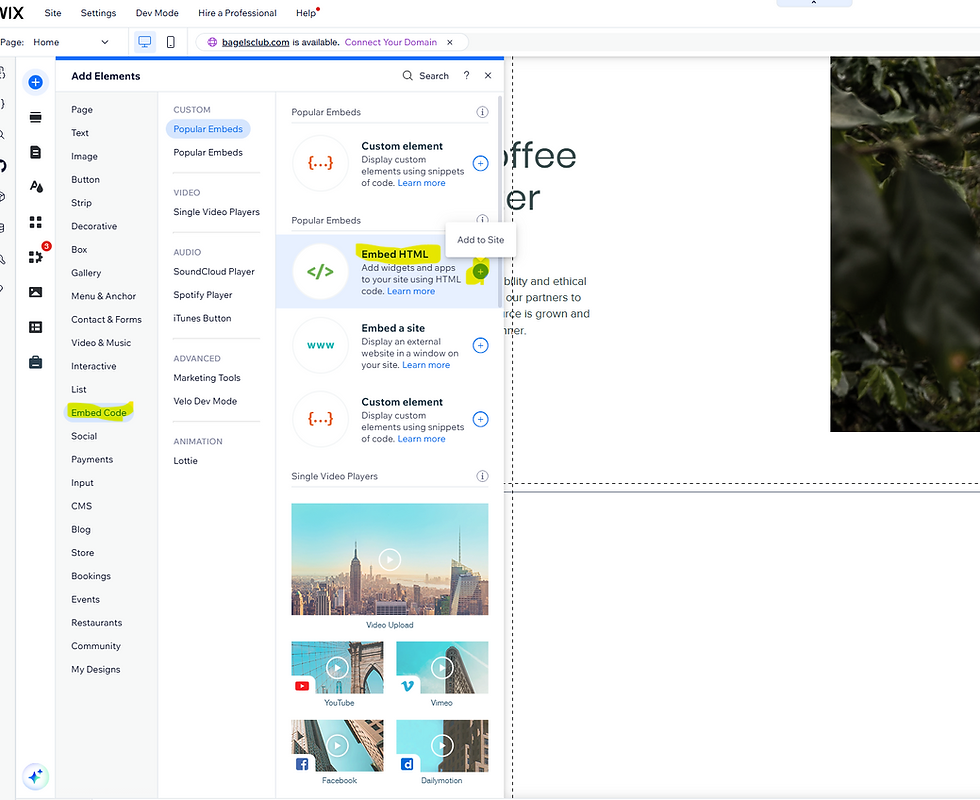
Insert the Base HTML: Paste this HTML code into the iframe:
<div id="map" style="width: 100%; height: 100%;"></div>
<script>
function initMap() {
const map = new google.maps.Map(document.getElementById("map"), {
center: { lat: 40.73061, lng: -73.935242 },
zoom: 12,
});
}
</script>
<script src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=initMap" async defer></script>
Replace YOUR_API_KEY with your actual Google Maps API key.
Connect Wix Velo Code: Use Wix Velo to dynamically load data into the map. Add a dataset or collection in your Wix Dashboard, such as a list of business locations, and retrieve this data via code.
Step 3: Display Dynamic Locations
Displaying dynamic locations on a Google Map allows you to showcase multiple points of interest, such as store locations, event venues, or service coverage areas, directly on your Wix website. By combining Wix Velo and Google Maps, you can automate the process of retrieving location data from a database collection and display it visually on the map. Here's a detailed walkthrough:
1. Create a Database Collection for Locations
To make your map dynamic, you first need to store the data for your locations. Use the Wix Content Manager to create and manage this collection.
Go to your Wix dashboard and navigate to Content Manager.
Click Create New Collection and name it something like Locations.
Add the following fields to your collection:
Name (Text): The name of the location (e.g., "Downtown Office").
Address (Text): The physical address (optional for display but not used by Google Maps directly).
Latitude (Number): The latitude coordinate of the location.
Longitude (Number): The longitude coordinate of the location.
Pro Tip: Use an online geocoding tool to generate the latitude and longitude for your addresses, or utilize the Google Maps Geocoding API for automation.
2. Populate Your Collection with Data
Add entries for each location in your collection. For example:
Name | Address | Latitude | Longitude |
Downtown Office | 123 Main St, City A | 40.73061 | -73.935242 |
Uptown Branch | 456 Elm St, City B | 40.748817 | -73.985428 |
Suburban Office | 789 Oak Ave, Suburb C | 40.712776 | -74.005974 |
This collection will serve as the source of data for your map.
3. Retrieve Location Data with Wix Velo
Now, use Wix Velo to fetch this data and send it to your Google Map. Below is a step-by-step process:
Add the HTML Element for Google Maps
Paste the following code into your HTML Embed element in the Wix Editor:
<div id="map" style="width: 100%; height: 100%;"></div>
<script>
let map; // Global map object
// Initialize the map
function initMap(lat = 40.73061, lng = -73.935242, zoom = 10) {
console.log("Initializing map...");
map = new google.maps.Map(document.getElementById('map'), {
center: { lat, lng },
zoom,
});
console.log("Map initialized with center:", { lat, lng }, "and zoom:", zoom);
}
// Add markers dynamically
function addMarkers(locations) {
console.log("Adding markers:", locations);
locations.forEach(location => {
const marker = new google.maps.Marker({
position: { lat: location.lat, lng: location.lng },
map: map,
title: location.name,
});
console.log("Marker added for:", location.name, "at", { lat: location.lat, lng: location.lng });
});
}
// Listen for messages from Wix
window.onmessage = (event) => {
console.log("Received message:", event.data);
const data = event.data;
if (data.type === 'updateMap') {
console.log("Updating map center to:", { lat: data.lat, lng: data.lng, zoom: data.zoom });
map.setCenter({ lat: data.lat, lng: data.lng });
map.setZoom(data.zoom);
if (data.locations) {
console.log("Locations provided for markers:", data.locations);
addMarkers(data.locations);
} else {
console.log("No locations provided for markers.");
}
}
};
// Load Google Maps API and initialize the map
const script = document.createElement('script');
script.src = `https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=initMap`;
script.async = true;
script.defer = true;
document.head.appendChild(script);
console.log("Google Maps API script loaded.");
</script>
This code dynamically creates markers based on your database entries.
Write Wix Velo Code to Fetch Data
Switch to the Code Editor in Wix and add the following Velo code to retrieve data from your Locations collection and pass it to the map.
import wixData from 'wix-data';
$w.onReady(() => {
console.log("Page is ready. Fetching location data...");
// Query the Locations collection
wixData.query('Locations') // Replace 'Locations' with your collection name
.find()
.then(results => {
console.log("Locations fetched from database:", results.items);
// New York coordinates
const latitude = 40.7128; // Latitude for New York
const longitude = -74.0060; // Longitude for New York
const zoomLevel = 12;
const locations = results.items.map(item => ({
lat: item.latitude,
lng: item.longitude,
name: item.name,
}));
console.log("Formatted locations for markers:", locations);
// Send data to the HTML Embed element
$w('#html1').postMessage({
type: 'updateMap',
lat: latitude,
lng: longitude,
zoom: zoomLevel,
locations: locations,
});
console.log("Message sent to HTML Embed:", {
type: 'updateMap',
lat: latitude,
lng: longitude,
zoom: zoomLevel,
locations: locations,
});
})
.catch(error => {
console.error("Error fetching location data:", error);
});
});
This code:
Queries the Locations Collection: Retrieves all location entries.
Formats Location Data: Extracts latitude, longitude, and names into a usable format.
Sends Data to the HTML Embed: Dynamically updates the map center and markers using the postMessage API.tMessage(locations) sends the data to the embedded map.
4.What You Should See
After completing the steps above, your map should look similar to this:
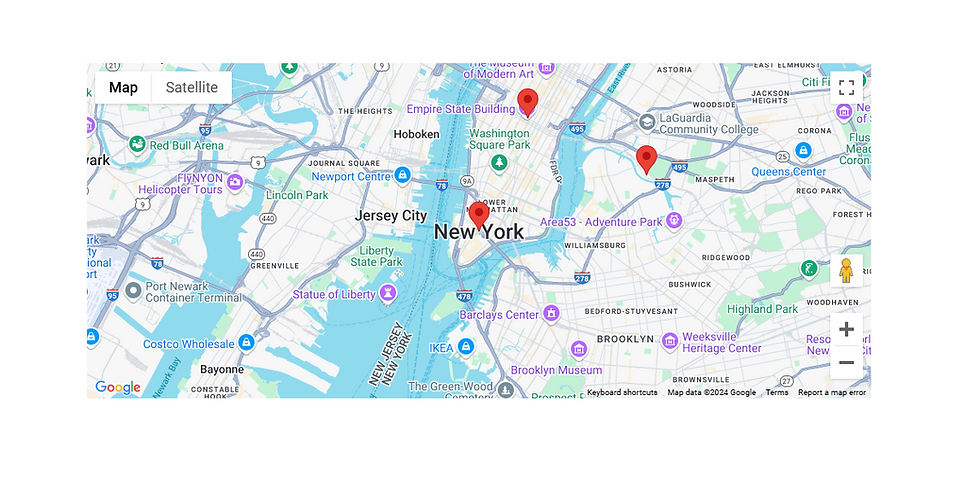
This map centers on New York and displays markers for the locations retrieved from your database. You can zoom in, pan around, and interact with the markers to view details about each location.
Conclusion: Google Maps Customization with Wix Velo:
By following this guide, you’ve learned how to dynamically center a Google Map and add markers based on your Wix database. Whether you're showcasing store locations, event venues, or points of interest, this integration provides a seamless way to enhance the functionality of your Wix site using Velo.
If you're interested in exploring more Wix Velo topics, check out our blog for additional tutorials on Velo integrations. From advanced database integrations to custom scripts, we cover a variety of topics to help you unlock the full potential of Wix Velo.
Need Help? Contact Us!
At CodeMasters, we specialize in delivering powerful, customized solutions for Wix websites. If you have any questions or need assistance with your Wix project, don’t hesitate to contact us here. Let us help you create a website that works exactly the way you need it to!